Using Cloudflare Workers to mediate between MinIO and Discord
MinIO's lack of support for webhook output formatted for quick messaging apps has annoyed me for a fair while now, and so today I finally snapped and got around to doing something about it. Much like my previous article, because I'm too lazy to spin up a proper dedicated process I'll be running it on a simple Cloudflare Worker (which also conveniently exposes a POST-able URL).
The first step is, of course, figuring out what kind of data I want in the first place. I manage a relatively low-traffic MinIO instance, so I'm not really interested in anything but put/delete activity in selected buckets. The first step (that is, after creating a blank Cloudflare Worker!) in obtaining this data is to set up an Event destination in the MinIO admin panel, with the endpoint URL set to your new CF Worker.
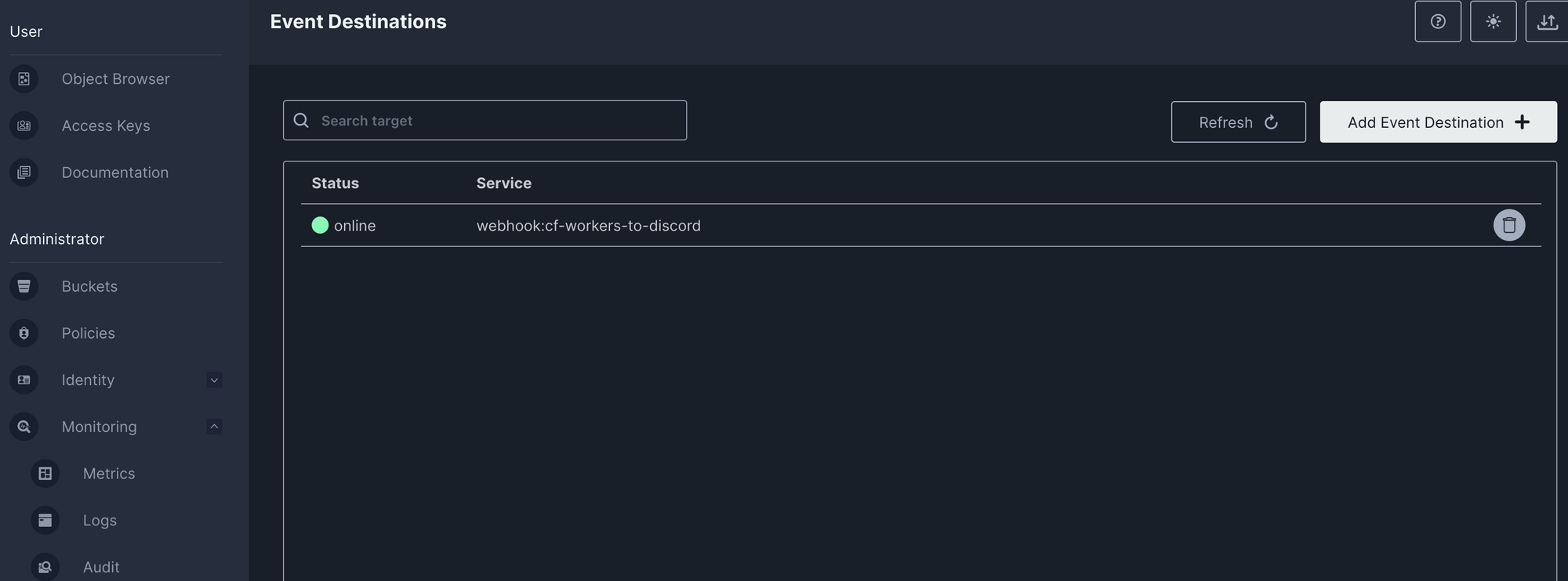
Then, to make use of this destination, you have to select a bucket and subscribe to its events, which lets you select a destination for the events to be sent to & which events will be logged (put & delete, in this case).
To understand what form the MinIO JSON payload comes in, I printed the request payload sent to my Worker. Here's a slightly reformatted (for better readability) version of what was sent after I uploaded a test file:
- EventName:
s3:ObjectCreated:Put
- Key:
misskey/watchtower.png
- Records:
- [0]:
- eventVersion:
2.0
- eventSource:
minio:s3
- awsRegion:
ap-northeast-2
- eventTime:
2025-02-03T07:30:12.289Z
- eventName:
s3:ObjectCreated:Put
- userIdentity:
- principalId:
admin
- requestParameters:
- principalId:
admin
- region:
ap-northeast-2
- sourceIPAddress:
45.87.213.216
- responseElements:
- x-amz-id-2:
dd9025bab4ad464b049177c95eb6ebf374d3b3fd1af9251148b658df7ac2e3e8
- x-amz-request-id:
1820A236C3BA10CF
- x-minio-deployment-id:
94aaa601-26fd-4283-a26b-5b3f890b51d7
- x-minio-origin-endpoint:
http://172.17.0.16:9000
- s3:
- s3SchemaVersion:
1.0
- configurationId:
Config
- bucket:
- name:
misskey
- ownerIdentity:
- principalId:
admin
- arn:
arn:aws:s3:::misskey
- object:
- key:
watchtower.png
- size:
36798
- eTag:
28a7de530041b8a89f964927afc5661a
- contentType:
image/png
- userMetadata:
- content-type:
image/png
- sequencer:
1820A236C3BDF33C
- source:
- host:
45.87.213.216
- port:
- userAgent:
MinIO (linux; amd64) minio-go/v7.0.83 MinIO Console/(dev)
For a quick, easily understandable notification to be posted in a Discord channel, I judged that only the following fields are necessary: EventName
, Key
, Records[0].eventTime
, Records[0].requestParameters.sourceIPAddress
, Records[0].s3.bucket.name
, Records[0].s3.object.size
, and Records[0].s3.object.contentType
.
From there, extracting the data is fairly standard JSON manipulation, so I won't bore you with the details. Of course, now the task of structuring the Discord payload lies ahead. I forgot to mention it in the previous article, but this reference for Discord webhook payload format was a great help in figuring out how I should proceed.
In this case, I decided to go with something like this:
const embedFields = [
{
name: "File Key",
value: fileKey,
inline: true,
},
{
name: "Bucket",
value: bucketName,
inline: true,
},
{
name: "Event Time",
value: eventTime,
inline: true,
},
{
name: "File Size (bytes)",
value: String(fileSize),
inline: true,
},
{
name: "Content Type",
value: contentType,
inline: true,
},
{
name: "Source IP",
value: sourceIP,
inline: true,
},
];
return {
username: "MinIO Webhook",
embeds: [
{
title: "MinIO Event",
description: `A \`${eventName}\` event occurred in bucket \`${bucketName}\`.`,
color: 15258703,
fields: embedFields,
},
],
};
Which, in Discord, looks something like this:
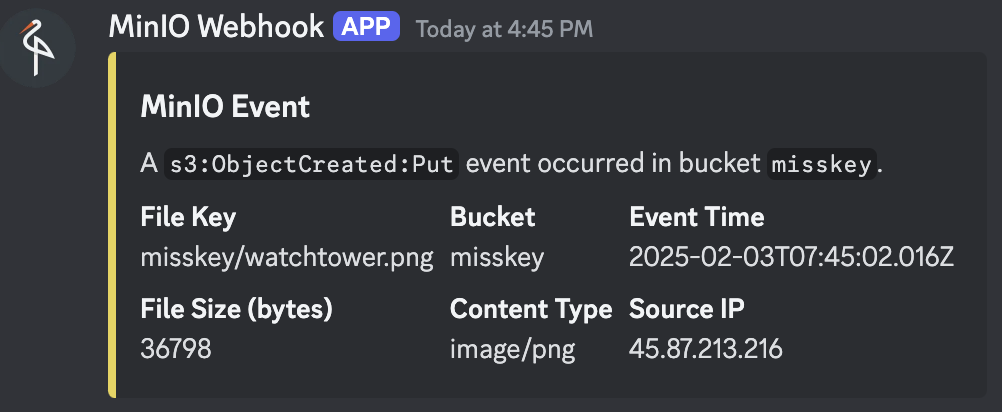
Neat! Obviously, this isn't practical if your subscribed bucket experiences extremely high amounts of traffic, but for a smaller-scale deployment like mine where I have full control over user access this suits me just fine.
Here's what you're probably here for- the full code of my Worker, ready for use once you fill in your Discord webhook URL:
addEventListener("fetch", (event) => {
event.respondWith(processRequest(event.request));
});
async function processRequest(request) {
if (request.method !== "POST") {
return new Response("Method Not Allowed", { status: 405 });
}
try {
const minioData = await request.json();
const discordPayload = createDiscordPayload(minioData);
const DISCORD_WEBHOOK_URL =
"<YOUR DISCORD WEBHOOK URL HERE>";
const discordResponse = await fetch(DISCORD_WEBHOOK_URL, {
method: "POST",
headers: { "Content-Type": "application/json" },
body: JSON.stringify(discordPayload),
});
if (!discordResponse.ok) {
return new Response(
`Discord returned a non-OK status: ${discordResponse.status}`,
{ status: 500 }
);
}
return new Response("OK", { status: 200 });
} catch (error) {
return new Response(error.toString(), { status: 500 });
}
}
function createDiscordPayload(minioData) {
const eventName = minioData.EventName || "Unknown Event";
const fileKey = minioData.Key || "Unknown Key";
const record =
Array.isArray(minioData.Records) && minioData.Records[0]
? minioData.Records[0]
: {};
const eventTime = record.eventTime || "N/A";
const sourceIP =
record.requestParameters && record.requestParameters.sourceIPAddress
? record.requestParameters.sourceIPAddress
: "N/A";
const bucketName =
record.s3 && record.s3.bucket && record.s3.bucket.name
? record.s3.bucket.name
: "N/A";
const objectDetails = record.s3 && record.s3.object ? record.s3.object : {};
const fileSize = objectDetails.size || "N/A";
const contentType = objectDetails.contentType || "N/A";
const embedFields = [
{
name: "File Key",
value: fileKey,
inline: true,
},
{
name: "Bucket",
value: bucketName,
inline: true,
},
{
name: "Event Time",
value: eventTime,
inline: true,
},
{
name: "File Size (bytes)",
value: String(fileSize),
inline: true,
},
{
name: "Content Type",
value: contentType,
inline: true,
},
{
name: "Source IP",
value: sourceIP,
inline: true,
},
];
return {
username: "MinIO Webhook",
embeds: [
{
title: "MinIO Event",
description: `A \`${eventName}\` event occurred in bucket \`${bucketName}\`.`,
color: 15258703,
fields: embedFields,
},
],
};
}
In a production environment, you might want to modify it to involve some sort of authorization, but I'll cross that bridge when I'm forced to.